Streamlining Evaluations: A Step-by-Step Tour of Assessment API

Introduction:
Imagine a world where choosing the perfect candidate for a job is like finding the right piece for a puzzle. Think about a school identifying the best students for its programs, or a workplace helping its employees grow. This magic happens with assessments – a kind of tool that makes sure everything fits just right. Today, we're diving into how these assessments work their magic in hiring, education, and workplaces. They're not just tests; they're the friendly wizards making sure everyone is in the right place and growing in the best way possible. Join us as we uncover how these assessments are the secret ingredients behind successful stories in the real world.
Fetching your Personal Access Key:
What is the X-access-key?
The X-access-key is like your digital signature, a distinct code that sets you apart. It's not just a key; it's your identifier, your way of saying, "Hey, I belong here!"
To initiate the utilisation of our API, you must obtain an X-access-key. Each Enterprise/Organisation user possessing a Skillwise for Owner account is provided with a distinctive X-access-key, accessible within their account settings. This key serves as a unique identifier and enables access to the API functionalities. The accompanying screenshot illustrates the process of locating and retrieving the X-access-key from your account.
Step 1 : Click on Create New Secret Key button from here
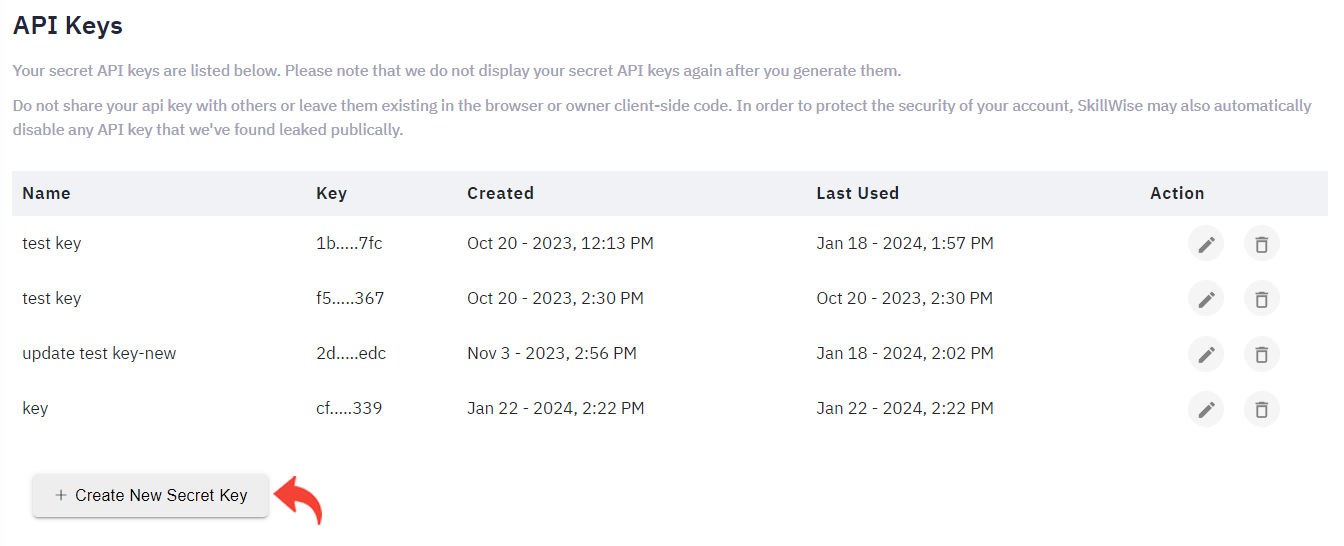
Step 2 : Give the desired key name and click on Create Secret Key button
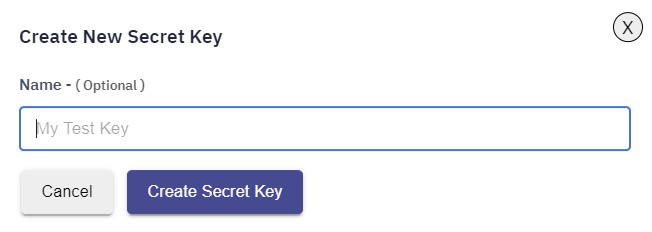
Step 3: Copy the key and store it in safe secured place as this key will not be displayed once you click on Done button
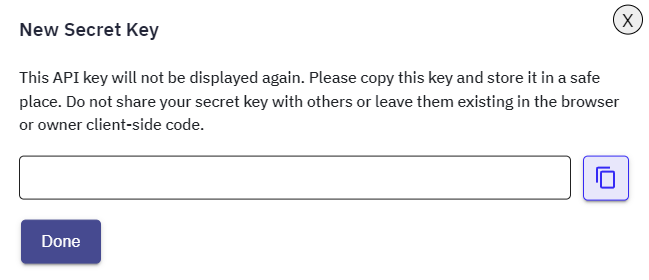
You have the flexibility to revoke any number of personal access keys and generate additional ones as needed. There is no restriction on the quantity of personal access keys that you can generate. Owner can also modify and delete the keys. Feel free to create as many as necessary to suit your requirements.
Step 1 : Create Assessment
Purpose
To create an assessment in your platform through an API request.
What are assessments?
Assessments enable you to evaluate systematically and analyse candidates during the hiring process. These assessments serve as valuable tools to identify an applicant's skills, competencies, and qualifications, helping your teams make informed hiring decisions. Not only hiring , assessments can also be used to evaluate candidate’s academic performances.
How to create assessments?
- To set up assessments, make sure you have a suitable 'test name' and a clear 'description' for the test.
- Assessments can be broken down into different sections, with each section containing multiple questions. When creating a test, it is required to have at least one section, and within that section, there must be a minimum of one question.
- For adding and editing specific sections and questions in the test, you can use dedicated section and question APIs, and we will provide further explanation on how to use them.
- In addition to the essential elements like test name, description, section, and question, the other settings are optional. You can find more details in the parameters table for reference.
Request URL
https://dev-test-backend-v2-edirdbg6ca-el.a.run.app/api/v1/access/test
Request Method
POSTconst axios = require('axios');
let data = {
"test_name": "Test1",
"description": "Description for test",
"sections": [{
"section_name": "section_name1",
"pass_percentage": 35,
"is_question_navigation": true,
"is_skip_question": true,
"duration": 30,
"questions": [{
"question": "How are you?",
"marks": 98,
"duration": 30,
"difficulty": 1,
"domain": "general",
"question_type": 1,
"ideal_answer": "answer",
"explanation": "explanation",
"tags": [
"reactjs",
"html"
],
"options": [{
"option": "437",
"is_solution": true
}]
}]
}]
};
let config = {
method: 'post',
maxBodyLength: Infinity,
url: 'https://dev-test-backend-v2-edirdbg6ca-el.a.run.app/api/v1/}access/test',
headers: {
'x-access-key': 'your_access_key',
'Content-Type': 'application/json'
},
data: data
};
axios(config)
.then((response) => {
console.log(JSON.stringify(response.data));
})
.catch((error) => {
console.log(error);
});
Parameters
Field Name | Type | Description | ||||||||||||||||||||||||||||||||||||
---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|
test_name * | String | The name or title of the assessment. | ||||||||||||||||||||||||||||||||||||
description * | String | A brief description of the assessment. | ||||||||||||||||||||||||||||||||||||
pass_percentage | Integer | The pass percentage required to pass the assessment. | ||||||||||||||||||||||||||||||||||||
invite_mail | Object | Email template for sending test invitations.
| ||||||||||||||||||||||||||||||||||||
advanced_settings | Object | Configure the advanced settings for the test, by default sets all to false.
| ||||||||||||||||||||||||||||||||||||
Proctor_settings | Object | Email template for sending test invitations.
| ||||||||||||||||||||||||||||||||||||
Sections * | Array | Sections represent the sections in the test
|
Step 2 : Create Section
Purpose
The "create section" API serves the purpose of adding a distinct section to an assessment on your platform, enhancing the structure and organisation of your tests.
Why Sections in Assessments?
Sections help break down assessments into meaningful parts, making it easier to evaluate candidates systematically. Each section can encompass multiple questions, allowing for a more detailed analysis of candidates' skills and competencies. This systematic approach aids in making well-informed hiring decisions and extends beyond hiring to evaluate academic performances.
How to Use the "Create Section" API:
After creating an assessment using the "create test" API and establishing the essential elements such as test name and description, the next step is to add sections. Each assessment should have at least one section, and within that section, there must be a minimum of one question. The "create section" API provides a dedicated method for incorporating and editing these specific sections.
Optional Settings and Parameters:
Similar to the "create test" API, the "create section" API allows for additional settings that enhance the customization of your assessments. These options include:
Section Name:
Assign a specific name to the section, providing clarity on its content.
Pass Percentage:
Set a pass percentage for the section, indicating the minimum score required for candidates to succeed.
Question Navigation:
Enable or disable question navigation, allowing candidates to move between questions.
Skip Question:
Determine whether candidates can skip questions within the section.
Duration:
Define the time limit for completing the section, ensuring a controlled assessment environment. Additionally, it's important to note that at least one question must be added to each section. Details on how to add questions will be explained in the "create question" API, ensuring that your assessments include the necessary components for a comprehensive evaluation. These settings offer flexibility in tailoring assessments to your specific needs, allowing you to create a more personalized and effective evaluation process.
Request URL
https://dev-test-backend-v2-edirdbg6ca-el.a.run.app/api/v1/access/section/{id}
Request Method
POSTconst axios = require('axios');
let data = {
"section_name": "section_name1",
"pass_percentage": 35,
"is_question_navigation": true,
"is_skip_question": true,
"duration": 30,
"questions": [{
"question": "How are you?",
"marks": 98,
"duration": 30,
"difficulty": 1,
"domain": "general",
"question_type": 1,
"ideal_answer": "answer",
"explanation": "explanation",
"tags": [
"reactjs",
"html"
],
"options": [{
"option": "437",
"is_solution": true
}]
}]
};
let config = {
method: 'post',
maxBodyLength: Infinity,
url: 'https://dev-test-backend-v2-edirdbg6ca-el.a.run.app/api/v1/access/invite/{id}',
headers: {
'x-access-key': 'your_access_key',
'Content-Type': 'application/json'
},
data: data
};
axios(config)
.then((response) => {
console.log(JSON.stringify(response.data));
})
.catch((error) => {
console.log(error);
})
Step 3 : Create Questions
Purpose
The ‘create question’ API is designed to facilitate the addition of specific questions to the sections within your assessment. This functionality enhances the granularity of your tests, allowing for detailed evaluation of candidates skills and knowledge.
Why Questions in Sections?
Questions serve as the building blocks of assessments, providing a means to evaluate candidates on specific topics. By adding questions to sections, you can systematically assess different aspects of a candidate's abilities, contributing to well-informed decision-making in hiring processes or academic evaluations.
How to Use the ‘Create Question’ API?
Following the creation of sections using the "create section" API, the next step is to add questions to these sections. Each section should have at least one question to ensure a comprehensive evaluation. The "create question" API offers a dedicated method for adding and editing these specific questions.For adding questions, use the 'id' of the test and the 'id' of the section from the 'create test' and 'create section' APIs. After getting the response, find the question 'id' labelled as '_id'. Remember this ID for future updates to questions.
Types of questions available :
Specify the type of question, choosing from various options.
MCQ (Single):
Multiple Choice Question with a single correct answer.
MCQ (Multiple):
Multiple Choice Question with multiple correct answers.
TITA:
"Type In The Answer" question where candidates need to type their response.
Essay:
Open-ended question requiring a more detailed written response.
Video:
Question that involves a video component.
Optional Settings and Parameters:
Similar to the "create test" and "create section" APIs, the "create question" API provides optional settings to customize your assessments. While details on these settings are not specified in this text, they can include parameters like question type, difficulty level, or multimedia attachments. Refer to the parameters table for more information.
Request URL
https://dev-test-backend-v2-edirdbg6ca-el.a.run.app/api/v1/access/question/{test_id}/{section_id}
Request Method
POSTconst axios = require('axios');
let data = {
"question": "How are you?",
"marks": 98,
"duration": 30,
"difficulty": 1,
"domain": "general",
"question_type": 1,
"ideal_answer": "answer",
"explanation": "explanation",
"tags": [
"reactjs",
"html"
],
"options": [{
"option": "437",
"is_solution": true
}]
};
let config = {
method: 'post',
maxBodyLength: Infinity,
url: 'https://dev-test-backend-v2-edirdbg6ca-el.a.run.app/api/v1/access/question/{test_id}/{section_id}',
headers: {
'x-access-key': 'your_access_key',
'Content-Type': 'application/json'
},
data: data
};
axios(config)
.then((response) => {
console.log(JSON.stringify(response.data));
})
.catch((error) => {
console.log(error);
});
Conclusion
By combining the "create test," "create section," and "create question" APIs, you can build a structured and detailed assessment that meets your specific needs, whether for hiring decisions or academic evaluations. These APIs offer flexibility and customization to enhance the effectiveness of your evaluation process.
Step 4 : Invite Candidates
Purpose
The "Send Invites" API allows you to send assessment invites to single candidates or multiple candidates simultaneously. It provides options to control whether candidates can re-appear for the assessment and whether invites can be resent to the candidates before they've appeared.
Single Invite Sending vs. Bulk Invite Sending:
Single Invite Sending:
You can use the API to send invites to individual candidates by providing their details in a single request.
Bulk Invite Sending:
You can send invites to multiple candidates simultaneously by providing an array of candidate details in a single request.
Sending Invites Methods:
Uploading CSV:
The API supports bulk invite sending by allowing you to upload a CSV file containing candidate details.
Customised Mail Body & Subject:
You have the flexibility to customise the email body and subject when sending invites, making the communication more personalised.
This API provides a versatile and efficient way to manage the invitation process for assessments, offering both single and bulk sending options with additional customization features.
Parameters:
Candidates
An array of objects containing candidate details required for sending test invites. This array can be used to send bulk or single invites.
Field:
email (required):
Candidate’s email ID to which the invite is sent.
name (required):
Candidate’s name (either first name or full name).
is_resend (required):
Boolean indicating whether invites can be resent to the candidates before they’ve appeared.
is_repeat (required):
Boolean indicating whether candidates can re-appear for the assessment.
Optional Settings and Parameters:
Similar to the "create test" and "create section" APIs, the "create question" API provides optional settings to customize your assessments. While details on these settings are not specified in this text, they can include parameters like question type, difficulty level, or multimedia attachments. Refer to the parameters table for more information.
Request URL
https://dev-test-backend-v2-edirdbg6ca-el.a.run.app/api/v1/access/invite/{id}
Request Method
POSTconst axios = require('axios');
let data = {
"candidates": [{
"email": "example@gmail.com",
"name": "rahul"
}],
"is_resend": true,
"is_repeat": true,
"subject": "updated invite mail subject"
};
let config = {
method: 'post',
maxBodyLength: Infinity,
url: ‘https: //dev-test-backend-v2-edirdbg6ca-el.a.run.app/api/v1/access/invite/{id}',
headers: {
'x-access-key': 'your_access_key',
'Content-Type': 'application/json'
},
data: data
};
axios(config)
.then((response) => {
console.log(JSON.stringify(response.data));
})
.catch((error) => {
console.log(error);
});
Step 5 : Get Results
You can obtain the result of a particular test or the result of all tests
- Result of a particular test
- Results of all tests
Result of a particular test :
Purpose
The Result API serves the purpose of retrieving detailed information related to a specific candidate's performance in an assessment. This API requires the candidate ID as input, and it provides comprehensive details that offer insights into the candidate's assessment results.
How to Use the Result API:
To utilize the Result API, you need to input the candidate ID, which can be obtained from the response of the Invite API. The candidate ID acts as a unique identifier for each candidate who has participated in the assessment.
Information Provided in the Result:
The API response includes a wealth of information, offering a detailed overview of the candidate's performance. This may encompass:
Scores:- Detailed breakdown of scores achieved in different sections or question categories.
Time Taken:- Duration taken by the candidate to complete the assessment.
Correct and Incorrect Answers:- A summary of correct and incorrect answers provided by the candidate.
Overall Assessment Outcome:- An overall evaluation or outcome of the assessment, indicating the candidate's proficiency or competency level.
Proctoring results:- It explains about various fraud that happened during the test.
Significance of the Candidate ID:
The candidate ID plays a crucial role as it uniquely associates the assessment results with a specific individual. This ID is obtained from the response of the Invite API when sending invitations to candidates.
Benefits of Using the Result API:
Individual Candidate Analysis:- Enables in-depth analysis of a candidate's performance on a granular level.
Data-Driven Decision Making:-Provides valuable data for making informed decisions in hiring or academic evaluation processes.
Tracking Progress:-Facilitates tracking and monitoring of candidate progress over multiple assessments.
Request URL
https://dev-test-backend-v2-edirdbg6ca-el.a.run.app/api/v1/access/result/{id}
Request Method
GETconst axios = require('axios');
let config = {
method: 'get',
maxBodyLength: Infinity,
url: 'https://dev-test-backend-v2-edirdbg6ca-el.a.run.app/api/v1/access/result/{id}’,
headers: {
'x-access-key': ‘your_access_key’,
'Content-Type': 'application/json'
}
};
axios(config)
.then((response) => {
console.log(JSON.stringify(response.data));
})
.catch((error) => {
console.log(error);
});
In summary, the Result API is a valuable tool for obtaining detailed insights into a candidate's performance, utilising the candidate ID obtained from the Invite API. It empowers organisations and educators to make informed decisions based on comprehensive assessment data.
Result of all tests :
Purpose
The Result of All Tests API serves the purpose of retrieving comprehensive details for all candidates who have participated in a specific test. To utilize this API, provide the 'test ID' obtained from the 'create test' API as a parameter. This API offers a consolidated view of the performance metrics and status of all candidates associated with the specified test.
Benefits and Use Cases:
Efficient Monitoring:- Provides an efficient way to monitor the overall progress and outcomes of a specific test.
Aggregated Statistics:-Consolidates statistics such as total invitations, completions, pass rates, etc., providing a holistic view.
Identification of Trends:- Helps in identifying trends, patterns, and areas for improvement based on the collective performance of candidates.
Example Use Case:
Imagine a hiring manager wants to review the outcomes of a coding test (specified by the 'test ID'). The Result of All Tests API can be used to retrieve information about the total number of candidates invited, the number of candidates who completed the test, the overall pass rate, and more.
This API is a powerful tool for obtaining aggregated insights into the performance of all candidates associated with a particular test, enabling organizations to make data-driven decisions and refine their assessment strategies.
Request URL
https://dev-test-backend-v2-edirdbg6ca-el.a.run.app/api/v1/access/invites/{id}
Request Method
GETconst axios = require('axios');
let config = {
method: 'get',
maxBodyLength: Infinity,
url: 'https://dev-test-backend-v2-edirdbg6ca-el.a.run.app/api/v1/access/invites/{id}?search=name_1,
headers: {
'x-access-key': 'your_access_key',
'Content-Type': 'application/json'
}
};
axios(config)
.then((response) => {
console.log(JSON.stringify(response.data));
})
.catch((error) => {
console.log(error);
});
Conclusion:
As we wrap up our journey through the impactful world of assessments, here's a simple yet powerful message: Imagine a platform where creating assessments is as easy as sending an invitation and viewing results. Whether you're an educational institution seeking to identify promising students, an office conducting internal evaluations, or a hiring platform striving to build exceptional teams – our platform is here for you.With seamless assessment creation, effortless candidate invitations, and a user-friendly results view, our platform simplifies the complex. It's not just a tool; it's your ally in shaping brighter futures. So, whether you're fostering academic excellence, nurturing workplace talent, or curating your dream team, our platform is the key to unlocking success. Join us in making assessments a breeze – because your journey to excellence starts here.